Kibor |
Отправлено: 30 Января, 2019 - 23:01:18
|

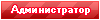
Full Member

Покинул форум
Сообщений всего: 160
Дата рег-ции: Дек. 2017
Репутация: 0
Карма 0

|
In this version, the refinement was the implementation of the ability to create functions responsible for capturing various messages.
Added getmessage operator
Functions created using this operator capture all messages received by modeless Cybor dialog boxes.
Such as WM_MOUSEMOVE, WM_MENUCOMMAND, WM_LBUTTONDOWN, WM_PAINT and many others.
This allows you to fully interact with the window.
Syntax:
Цитата: function getmessage(0, WM_LBUTTONDOWN)//Отлавливаем клик левой кнопки мыши
{
beep(2500, 100);
}
Functions getmessage call is not necessary. They themselves are called when a window receives a message. In this case, this function will be called by itself if you click the left mouse button in the non-modal Cybor dialog. To specify a dialog in which to catch messages and which message to catch in brackets we specify 2 parameters.
The first is the dialog number created using non-modal dialog boxes
The second is a constant or numeric value of something constant, corresponding to the type of message to which the code should be executed in this function.
When you receive a message, the structure becomes available:
Цитата: message.x - the coordinate of the mouse X
message.y - the coordinate of the mouse Y
message.wparam - defines additional information about the message. The exact value depends on the value of the message structure member.
message.lparam - defines additional information about the message. The exact value depends on the value of the message structure member.
Below is an example of how using these functions to determine the selected menu item created in the non-modal Cybor dialog, catch messages moving the mouse over the dialog, click the left mouse button and refresh the window.
CODE:external(INT, "CreateMenu", "CreateMenu", "User32.dll" );
external(INT, "CreatePopupMenu", "CreatePopupMenu", "User32.dll" );
external(INT, "AppendMenu", "AppendMenuA", "User32.dll" );
external(INT, "SetMenuInfo", "SetMenuInfo", "User32.dll" );
external(INT, "SetMenu", "SetMenu", "User32.dll" );
external(INT, "CreateWindow", "CreateWindowExA", "User32.dll" );
external(INT, "TrackPopupMenu", "TrackPopupMenu", "User32.dll" );
//Сожание меню
int MENUINFO[7]={28, 16, 134217728, 0, 0, 0, 0};
int HMENU, HMENU2, HMENU3;
HMENU2=CreatePopupMenu();
AppendMenu(HMENU2, 16, 1, "Новый" );
AppendMenu(HMENU2, 16, 2, "Открыть" );
AppendMenu(HMENU2, 16, 3, "Сохранить" );
AppendMenu(HMENU2, 16, 4, "Сохранить как" );
HMENU3=CreatePopupMenu();
AppendMenu(HMENU3, 16, 5, "Вырезать" );
AppendMenu(HMENU3, 16, 6, "Копировать" );
HMENU=CreateMenu();
AppendMenu(HMENU, 16, HMENU2, "Файл" );
AppendMenu(HMENU, 16, HMENU3, "Правка" );
//Создание всплывающего меню
int vHMENU, vHMENU2, result;
vHMENU=CreatePopupMenu();
AppendMenu(vHMENU, 0, 111, "MessageBox" );
AppendMenu(vHMENU, 0, 222, "Findimage" );
vHMENU2=CreatePopupMenu();
AppendMenu(vHMENU2, 0, 333, "25" );
AppendMenu(vHMENU2, 0, 444, "50" );
AppendMenu(vHMENU2, 0, 555, "75" );
AppendMenu(vHMENU2, 0, 666, "100" );
AppendMenu(vHMENU, 16, vHMENU2, "Прозрачность" );
createdialog(0);
showdialog(0, "Диалог",100, 100, 300, 300, 1, 1);
while(window ("Диалог")==0)sleep(10);
win w=window ("Диалог");
SetMenuInfo(HMENU, address(#MENUINFO[0]));
SetMenu(formatwi(w), HMENU);
//Создание кнопок
int b1=CreateWindow(0, "BUTTON", "Первая кнопка", 1342177280, 10, 10, 120, 30, formatwi(w), 0, 0, 0);
int b2=CreateWindow(0, "BUTTON", "Ворая кнопка", 1342177280, 10, 50, 120, 30, formatwi(w), 0, 0, 0);
loop()
{
w=window ("Диалог");
textout(1, w.left, w.top, " Не обновляли ", 0);
sleep(1);
}
function Простая_функция()//В ней пересчитаем на экранные координаты
{
textout(0, message.x+w.leftclient, message.y+w.topclient, " "+format(message.x)+" "+format(message.y), 1);
}
function getmessage(0, WM_MOUSEMOVE)//Отлавливаем перемещешие мыши над окном
{
Простая_функция();//Перейдем в другую функцию
}
function getmessage(0, WM_COMMAND)
{
if (message.lparam==b1)messagebox ("Первая кнопка");
if (message.lparam==b2)messagebox ("Ворая кнопка");
}
function getmessage(0, WM_MENUCOMMAND)//Отлавливаем клик по пункту меню
{
if (message.lparam==HMENU2)//идентификатор меню "Файл"
{
if (message.wparam==0)messagebox ("Новый");
if (message.wparam==1)messagebox ("Открыть");
if (message.wparam==2)messagebox ("Сохранить");
if (message.wparam==3)messagebox ("Сохранить как");
}
if (message.lparam==HMENU3)//идентификатор меню "Правка"
{
if (message.wparam==0)messagebox ("Вырезать");
if (message.wparam==1)messagebox ("Копировать");
}
}
function getmessage(0, WM_RBUTTONDOWN)//Отлавливаем клик правой кнопки мыши всплывающее меню
{
w=window ("Диалог" );
result=TrackPopupMenu(vHMENU, 256, message.x+w.leftclient, message.y+w.topclient, 0, formatwi(w), 0 ); // вывести меню
if(result==111)messagebox("Выбран пункт MessageBox" );
if(result==222)messagebox("Выбран пункт Findimage" );
if(result==333)transparency(25, w);
if(result==444)transparency(50, w);
if(result==555)transparency(75, w);
if(result==666)transparency(100, w);
}
function getmessage(0, WM_LBUTTONDOWN)//Отлавливаем клик левой кнопки мыши
{
beep(2500, 100);
}
function getmessage(0, 15)//WM_PAINT Отлавливаем обновление окна
{
textout(1, w.left, w.top, " Обновили окно ", 0);
sleep(100);
}
----- The Visual Code Editor is Kibor. Creating bots without knowledge of programming.
Learning function for recognizing text.
----- |
|
|
Kibor |
Отправлено: 01 Февраля, 2019 - 15:51:11
|

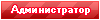
Full Member

Покинул форум
Сообщений всего: 160
Дата рег-ции: Дек. 2017
Репутация: 0
Карма 0

|
Example of creating and processing a slider and replacing text in static
CODE:external(INT, "CreateWindow", "CreateWindowExA", "User32.dll" );
external(INT, "SetScrollRange", "SetScrollRange", "User32.dll" );
external(INT, "SetScrollPos", "SetScrollPos", "User32.dll" );
external(INT, "SetWindowText", "SetWindowTextA", "User32.dll" );
createdialog(0);
showdialog(0, "Диалог",100, 100, 300, 300, 1, 1);
while(window ("Диалог")==0)sleep(10);
win w=window ("Диалог");
//Создание кнопок
int b1=CreateWindow(0, "BUTTON", "Первая кнопка", 1342177280, 10, 10, 120, 30, formatwi(w), 0, 0, 0);
int b2=CreateWindow(0, "BUTTON", "Ворая кнопка", 1342177280, 10, 50, 120, 30, formatwi(w), 0, 0, 0);
int s1=CreateWindow(0, "scrollbar", 0, 1342177280, 10, 100, 200, 20, formatwi(w), 0, 0, 0);
int hText = CreateWindow(0, "STATIC", "",1342177280, 10, 150, 100, 15, formatwi(w), 0, 0, 0);
int min=0, max=100, pos=95;
SetScrollRange(s1,2,min,max,1);
SetScrollPos(s1,2,pos,1);
Изменить_текст_и_прозрачность();
loop()sleep(1);
function Изменить_текст_и_прозрачность()
{
SetWindowText(hText, format(pos));
transparency(pos, w);
}
function getmessage(0, WM_COMMAND)
{
if (message.lparam==b1)messagebox ("Первая кнопка");
if (message.lparam==b2)messagebox ("Ворая кнопка");
}
function getmessage(0, WM_HSCROLL)
{
if (message.lparam==s1)
{
if (message.wparam==1 || message.wparam==3)//SB_LINERIGHT
{
pos=pos+10;
if (pos>max)pos=max;
SetScrollPos(s1,2,pos,1);
Изменить_текст_и_прозрачность();
return;
}
if (message.wparam==0 || message.wparam==2)//SB_LINELEFT
{
pos=pos-10;
if (pos<min)pos=min;
SetScrollPos(s1,2,pos,1);
Изменить_текст_и_прозрачность();
return;
}
///////////
if (loword(message.wparam)==5)//Зажали и двигаем
{
pos=pos-(pos-hiword(message.wparam));
if (pos>max)pos=max;
if (pos<min)pos=min;
SetScrollPos(s1,2,pos,1);
Изменить_текст_и_прозрачность();
}
}
}
//
----- The Visual Code Editor is Kibor. Creating bots without knowledge of programming.
Learning function for recognizing text.
----- |
|
|
Kibor |
Отправлено: 02 Февраля, 2019 - 19:05:39
|

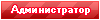
Full Member

Покинул форум
Сообщений всего: 160
Дата рег-ции: Дек. 2017
Репутация: 0
Карма 0

|
Added gethwnd function
Returns the HWND of the created dialog box using the createdialog, for use in WinApi.
The advantage of getting the HWND in this way is that it is received when the WM_CREATE message is called.
In the handler of this message it is necessary to create all the necessary controls.
This function can be called without creating message handlers immediately after the createdialog. In this case, do not wait for the creation of the window and getting the pointer using the window.
Syntax:
Parameter - the number of the dialogue (0 or 1 or 2)
Example of using and creating a button in the WM_CREATE handler
CODE:external(INT, "CreateWindow", "CreateWindowExA", "User32.dll" );
int b;
//////////
createdialog(0);
showdialog(0, "Диалог", 258, 234, 250, 300, 1, 1);
//////////
loop() sleep(10);//Убрать после теста
function getmessage(0, WM_CREATE)
{
b=CreateWindow(0, "BUTTON", "Первая кнопка", 1342177280, 10, 10, 120, 30, gethwnd(0), 0, 0, 0);
}
function getmessage(0, WM_COMMAND)
{
if (message.lparam==b)messagebox ("Первая кнопка");
}
----- The Visual Code Editor is Kibor. Creating bots without knowledge of programming.
Learning function for recognizing text.
----- |
|
|
Kibor |
Отправлено: 05 Февраля, 2019 - 06:07:15
|

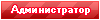
Full Member

Покинул форум
Сообщений всего: 160
Дата рег-ции: Дек. 2017
Репутация: 0
Карма 0

|
CODE:#define WS_VISIBLE 268435456
#define WS_CHILD 1073741824
#define BS_AUTOCHECKBOX 3
external(INT, "CreateWindow", "CreateWindowExA", "User32.dll" );
external(INT, "CreateFont", "CreateFontA", "Gdi32.dll" );
external(INT, "SendMessage", "SendMessageA", "User32.dll" );
int b1, b2;
createdialog(0);
showdialog(0, "Диалог",100, 100, 300, 300, 1, 1);
loop()sleep(1);
function getmessage(0, WM_COMMAND)
{
if (message.lparam==b1)messagebox ("кнопка");
if (message.lparam==b2)messagebox ("галка");
}
function getmessage(0, WM_CREATE)
{
int h_font = CreateFont(-13, 0, 0, 0, 400, 0, 0, 0, 0, 0, 0, 0, 0, "Times New Roman");
b1=CreateWindow(0, "BUTTON", "Кнопка", WS_VISIBLE | WS_CHILD, 10, 10, 120, 30, gethwnd(0), 0, 0, 0);
b2=CreateWindow(0, "BUTTON", "Галка", WS_VISIBLE | WS_CHILD | BS_AUTOCHECKBOX, 10, 50, 120, 30, gethwnd(0), 0, 0, 0);
SendMessage(b1, WM_SETFONT, h_font, 1);
}
----- The Visual Code Editor is Kibor. Creating bots without knowledge of programming.
Learning function for recognizing text.
----- |
|
|
|